I decided to use Spring Boot for my business, and I had to input the concept of annotation into my head, which had only the contents of Java training for new graduates long ago.
It was really easy to understand http://www.atmarkit.co.jp/ait/articles/1105/19/news127.html
Especially in this example
Annotation means "annotation". I used Javadoc comments to explain to developers what a program looks like, but there are times when I want to tell the compiler and execution environment what a program looks like.
As you can see from the concrete example described later, in Java, you can suppress the warning message output by the compiler or change the behavior of the program depending on the execution environment by writing annotations in the program.
I referred to here for the usage at the time of execution. https://www.qoosky.io/techs/390aad36f8
java version 1.8.0_181 Java(TM) SE Runtime Environment (build 1.8.0_181-b13) Java HotSpot(TM) 64-Bit Server VM (build 25.181-b13, mixed mode)
src └test └java └annotation ├ Main.java └ MethodInformation.java
Let's create and set an annotation type as just a marker.
MethodInformation.java
package test.java.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Target;
//By adding Target annotation, you can specify "What is the annotation attached to?"
@Target(ElementType.TYPE)
public @interface MethodInformation {
}
Main.java
package test.java.annotation;
@MethodInformation
public class Main {
public static void main(String[] args) {
System.out.println("Hello World!");
}
}
Please here
public enum ElementType { /** Class, interface (including annotation type), or enum declaration */ TYPE, /** Field declaration (includes enum constants) */ FIELD, /** Method declaration */ METHOD, /** Formal parameter declaration */ PARAMETER, /** Constructor declaration */ CONSTRUCTOR, /** Local variable declaration */ LOCAL_VARIABLE, /** Annotation type declaration */ ANNOTATION_TYPE, /** Package declaration */ PACKAGE, /** * Type parameter declaration * * @since 1.8 */ TYPE_PARAMETER, /** * Use of a type * * @since 1.8 */ TYPE_USE }
## Run
It is output without any problem. Since it is an annotation that does nothing in particular, there is no change in the output content.
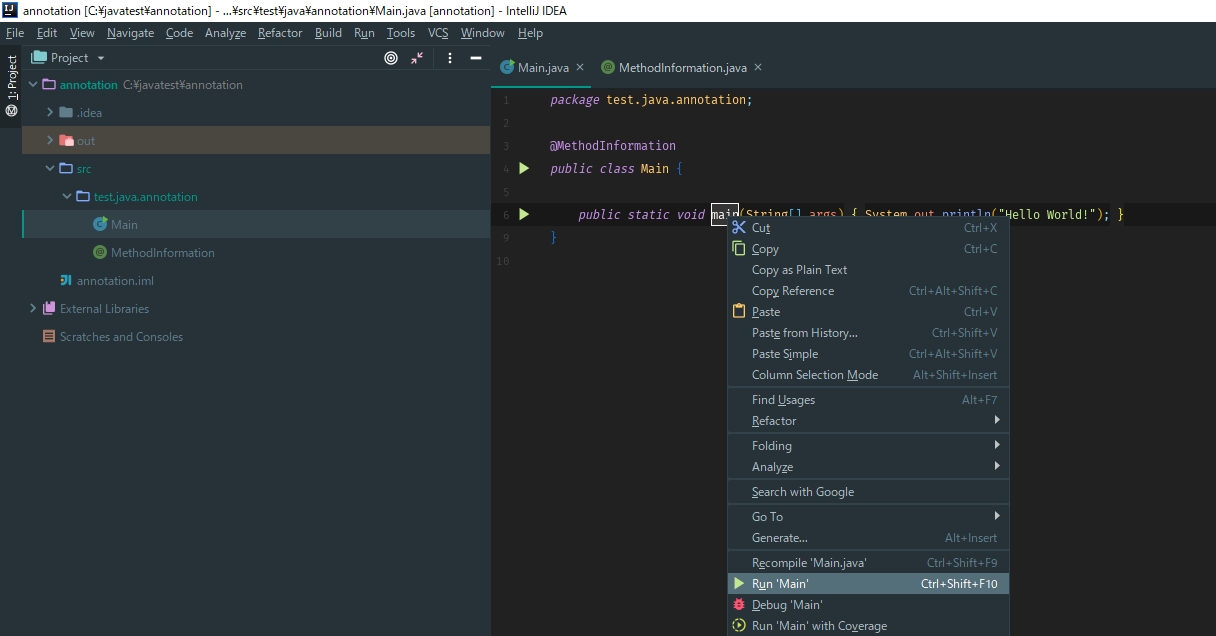
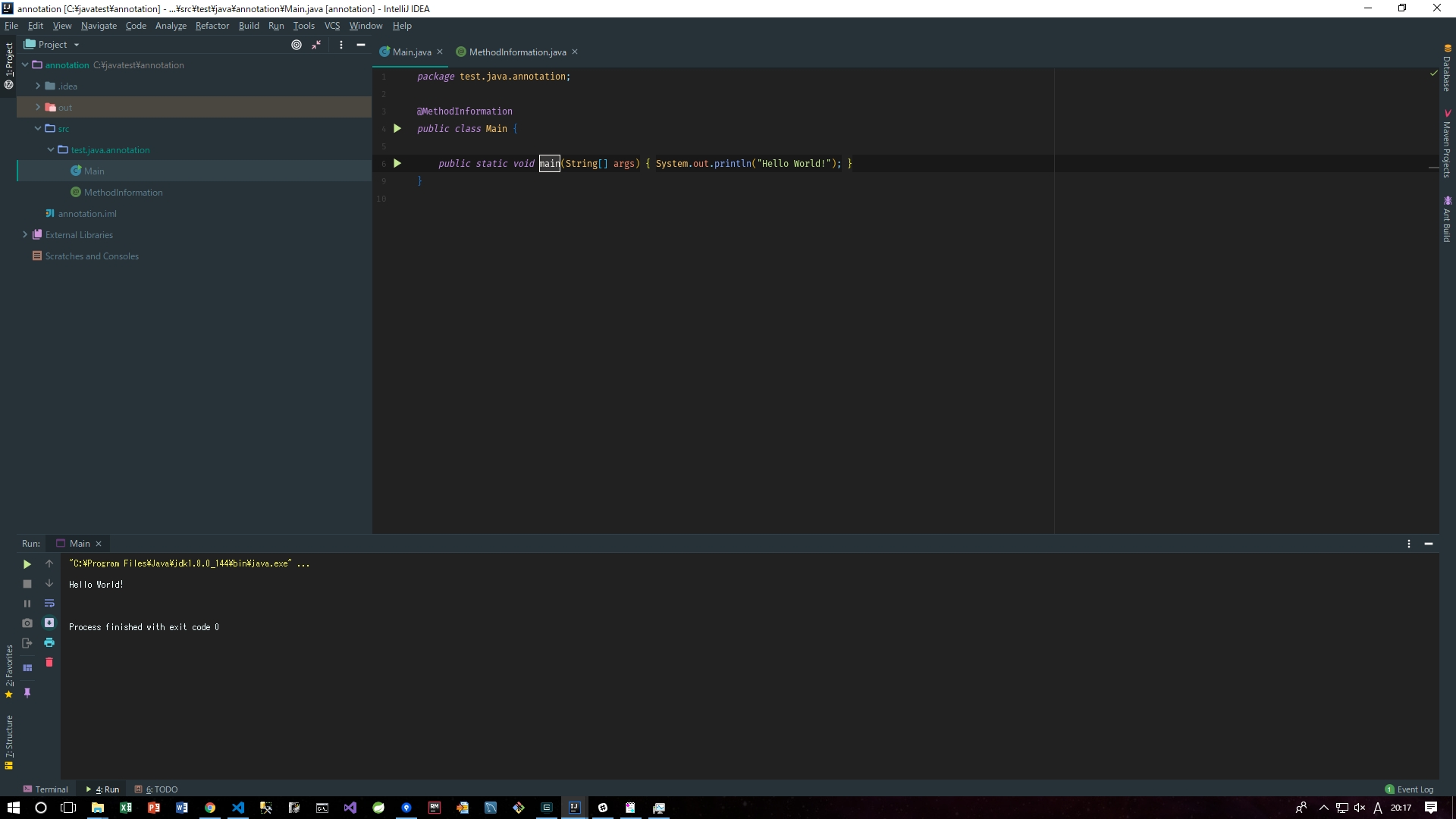
## Try to make annotations that can only be attached to methods
#### **`MethodInformation.java`**
```java
package test.java.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Target;
@Target(ElementType.METHOD) //Can only be attached to methods
public @interface MethodInformation {
}
It ’s a disturbing air.
Will fail safely
MethodInformation.java
package test.java.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME) //It is necessary to retain annotation information even when compiling → executing
public @interface MethodInformation {
}
Main.java
package test.java.annotation;
@MethodInformation
public class Main {
public static void main(String[] args) {
Main mainInstance = new Main();
Class clazz = mainInstance.getClass();
//Get the type of annotation set in the Main class
System.out.println(clazz.getAnnotation(MethodInformation.class));
System.out.println("Hello World!");
}
}
Please here
public enum RetentionPolicy { /** * Annotations are to be discarded by the compiler. */ SOURCE, /** * Annotations are to be recorded in the class file by the compiler * but need not be retained by the VM at run time. This is the default * behavior. */ CLASS, /** * Annotations are to be recorded in the class file by the compiler and * retained by the VM at run time, so they may be read reflectively. * * @see java.lang.reflect.AnnotatedElement */ RUNTIME }
You can see that @ test.java.annotation.MethodInformation () is output.
Create something called an annotation element and try to get its value
MethodInformation.java
package test.java.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
public @interface MethodInformation {
String testString();
int testInt();
}
Main.java
package test.java.annotation;
@MethodInformation(testString = "this is test", testInt = 12345)
public class Main {
public static void main(String[] args) {
Main mainInstance = new Main();
Class clazz = mainInstance.getClass();
MethodInformation methodInformation = (MethodInformation)clazz.getAnnotation(MethodInformation.class);
//Defined by method in annotation type
System.out.println(methodInformation.testString());
System.out.println(methodInformation.testInt());
System.out.println("Hello World!");
}
}
I was able to see the set value safely
MethodInformation.java
package test.java.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@MethodInformation(testString = "this is test double", testInt = 1234512345) //Annotate yourself
public @interface MethodInformation {
String testString() default "test";
int testInt() default 1234;
}
Main.java
package test.java.annotation;
@MethodInformation
public class Main {
public static void main(String[] args) {
Main mainInstance = new Main();
Class clazz = mainInstance.getClass();
MethodInformation methodInformation = (MethodInformation)clazz.getAnnotation(MethodInformation.class);
System.out.println(methodInformation.testString());
System.out.println(methodInformation.testInt());
System.out.println("Hello World!");
}
}
You can see that it has not been overwritten (the default value of the annotation is included). I feel a little strange.
Recommended Posts