There is a smart remote control called Nature Remo. I have been using it since last year because I can operate home appliances from smartphone apps and Google Home. This Remo is loaded with sensors that allow you to get temperature, humidity, illuminance, and human movement from the API (Remo mini is temperature only). This time, I would like to complete it only in the cloud using Saas of Azure to the point where I hit this API and collect data.
--No maintenance required --Can be built almost free of charge (a few yen will be charged to the storage account) --Data can be checked on the go
I thought it would be easier to build it using a Raspberry Pi, but sometimes my home network was cut off, so I thought about checking it from the outside and did it in the cloud.
Since Functions is used in Python this time, you cannot edit the code on the portal. Deploy from VVScode to Azure. Therefore, prepare VScode and the following extensions.
VScode extension
Azure Functions Core Tool https://docs.microsoft.com/ja-jp/azure/azure-functions/functions-run-local?tabs=windows%2Ccsharp%2Cbash
After installing the above extension, press F1 on VScode and press Azure: sign in. If you sign in on the opened browser, you can sign in to Azure with VScode.
CosmosDB Create Cosmos DB. This can be done from the portal or deployed from VScode. When creating with VScode
https://docs.microsoft.com/ja-jp/azure/azure-functions/functions-integrate-store-unstructured-data-cosmosdb?tabs=javascript
Cosmos DB has a free range, and you can use 400RU / sec and 5GB of storage for free as described in the FAQ below. https://azure.microsoft.com/pricing/details/cosmos-db/
Functions
Functions will be built from VScode this time because it is built in Python.
You now have a local project and function template.
First, make sure that you can hit Remo's API in Python normally. I used the Python code in the following article. Get an access token and make sure you can hit the API locally. As noted in this article, be careful not to leak your API key. Home appliances can be operated by others via API as much as you want. https://qiita.com/sohsatoh/items/b710ab3fa05e77ab2b0a
The result will be the following json. Since Remo mini is used, only the temperature is output in the item `te``` in
`newest_event```.
[
{
"name": "Remomini",
"id": <your remo id>,
"created_at": "2019-07-21T13:01:54Z",
"updated_at": "2020-04-29T05:18:05Z",
"mac_address": <your remo mac address>,
"serial_number": <your remo serial number>,
"firmware_version": "Remo-mini/1.0.92-g86d241a",
"temperature_offset": 0,
"humidity_offset": 0,
"users": [
{
"id": <your id>,
"nickname": <your nickname>,
"superuser": true
}
],
"newest_events": {
"te": {
"val": 23,
"created_at": "2020-04-30T05:10:10Z"
}
}
}
]
created_at
Date and time when remo was set,updated_at
Date and time when remo was updated(Will it be updated automatically?)It seems like.
This time I want to know `newest_event```, so I will send the contents and the time when I hit the API to Cosmos DB. This
created_at``` is different from the time you hit the API, as ``
newest_events` `` seems to be a specification that is updated when the sensor value changes.
I will put this code in the template code of timetrigger.
__init__.py
import datetime
import logging
import json
import requests
import azure.functions as func
def main(mytimer: func.TimerRequest, doc: func.Out[func.Document]) -> func.HttpResponse:
utc_timestamp = datetime.datetime.utcnow().replace(
tzinfo=datetime.timezone.utc).isoformat()
if mytimer.past_due:
logging.info('The timer is past due!')
apikey= "<your remo API key>"
# get JSON
headers = {
'accept' : 'application/json',
'Authorization' : 'Bearer ' + apikey ,
}
response = requests.get('https://api.nature.global/1/devices', headers= headers, verify=False)
rjson = response.json()
res_json = rjson[0]["newest_events"]["te"]
res_json["request_time"]= utc_timestamp
res_json = json.dumps(res_json,indent=2)
logging.info('Python timer trigger function ran at %s', utc_timestamp)
doc.set(func.Document.from_json(res_json))
mytimer: func.TimerRequest is azure.Timer from functions module
, ``` Doc: func.Out [func.Document]` `` means the output of azure.functions.
You can output by setting a value in the variable `` `doc``` that you just set. Describe that the variable ``` doc``` is the value to be sent to Cosmos DB in` `function.json``` generated when the function is created.
## Function app settings
1. Press F1 to bring up the command terminal, type binding and select Azure Functions: Add binding. (You can also right-click the function you just created locally from the Azure logo in the sidebar.)
2. Select the function you just created.
3. This time it is an output, so select out.
4. Select Azure Cosmos DB.
5. Enter the variable name to be used in the function. This time, let's say `` `doc```.
6. Enter the database name in Cosmos DB. This time, let's say `` `home` ``.
7. Enter the collection name in the database. This time, set it to ``` temperature``.
8. Set `` `true``` as to whether to create the DB if it does not exist.
9. You will be asked about the settings of the local environment, so create it with `` `Create new local app setting` ``.
10. You will be asked for a database account, select the `` `home``` you created.
11. Partition key is optional, so let's go through.
! Database name must be globally unique, so use any name
This will add the following to function.json:
{
"type": "cosmosDB",
"direction": "out",
"name": "doc",
"databaseName": "home",
"collectionName": "temperature",
"createIfNotExists": "true",
"connectionStringSetting": "cielohome_DOCUMENTDB"
}
You have now set the output. The Cosmos DB connectionString is listed in `` `local.settings.json``` in the same directory.
"cielohome_DOCUMENTDB": "AccountEndpoint=https://hogehoge.documents.azure.com:443/;AccountKey=fugafuga;"
## Test locally
Get the modules you need to test locally. This time, the required modules are ```azure-functions```, `` `requests```, so describe them in requirements.txt in the same directory.
azure-functions requests
Get this with ``` pip install requirements.txt```.
You can debug by pressing F5.
## Deploy to Functions
1. Press F1 to bring up the command terminal, search for deploy and select ```Azure Functions: deploy to function app .... `` `.
2. Select `` `Create new Function App in Azure (Advanced)` ``
3. Enter any resource name. (Globally unique name)
4. Select Python 3.7.
5. Select Coonsumption. It is pay-as-you-go. Functions also has a free frame, so basically you will not be charged if it is about once every 5 minutes.
6. Select a resource group. Use the same resource group as Cosmos DB. Different ones are fine. Note that the Python app will not work unless the resource group is made on Linux.
7. Select a storage account.
8. Select Application insights. It is Saas who sees the log.
Deployment is now complete.
## Check data with Cosmos DB
After deploying the function, let's take a look at the data.
Check the Cosmos DB in the sidebar by clicking the Azure logo on VScode. When you open the database, it is documented in ``` temperature```.
"val": 24.2, "created_at": "2020-05-02T13:49:12Z", "request_time": "2020-05-02T14:00:00.007771+00:00"
That's all there is to it.
## Summary
You can now store your data in Azure using Functions and Cosmos DB. Next time, I would like to visualize this data.
## reference
https://docs.microsoft.com/ja-jp/azure/azure-functions/functions-integrate-store-unstructured-data-cosmosdb?tabs=javascript
https://docs.microsoft.com/ja-jp/azure/azure-functions/
https://docs.microsoft.com/ja-jp/azure/azure-functions/functions-reference-python
<a target="_blank" href="https://www.amazon.co.jp/gp/product/B07CWNLHJ8/ref=as_li_tl?ie=UTF8&camp=247&creative=1211&creativeASIN=B07CWNLHJ8&linkCode=as2&tag=shirou463-22&linkId=fcd34229fcd07 > Nature Smart Remote Control Nature Remo mini Home Appliance Control Amazon Alexa / Google Home / Siri Compatible GPS Linked Temperature Sensor Remo-2W1 </a> <img src = "// ir-jp.amazon-adsystem.com/e/ir?t = shirou463-22 & l = am2 & o = 9 & a = B07CWNLHJ8 "width =" 1 "height =" 1 "border =" 0 "alt =" "style =" border: none! Important; margin: 0px! Important; "/>
## bonus
There is a software called Power BI Desktop from Microsoft, so when I tried to visualize it easily, it looked like this. It's easy because it's easy to link with Azure.
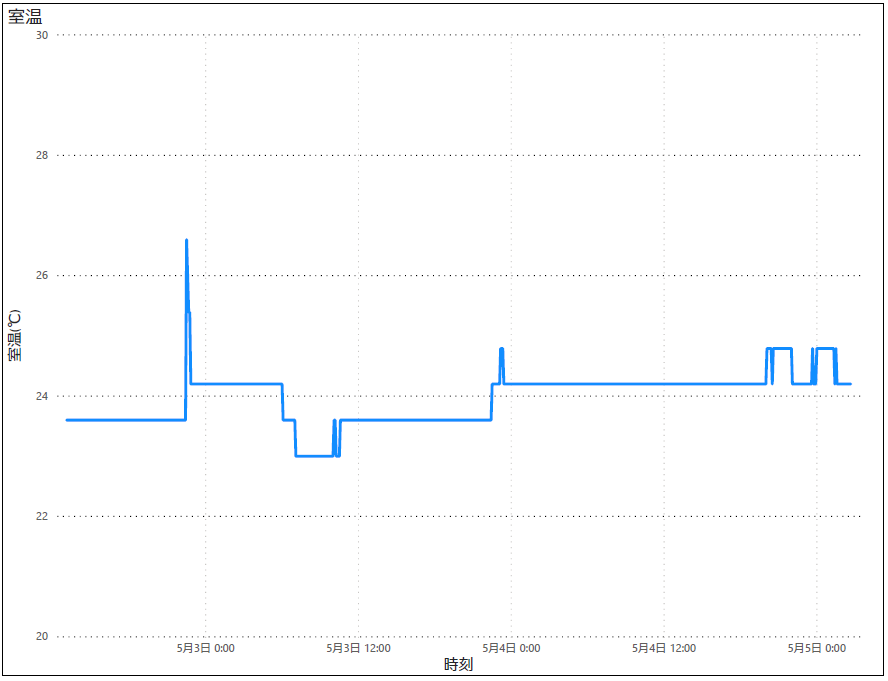
Recommended Posts