Automatic login to ServiceNow with Selenium Web Driver
◆ Overview
- I tried to log in to ServiceNow automatically using Selenium WebDriver, which is used for Web UI test automation.
◆ Operating environment
- OS: Windows10
- Python version: 3.8.0
◆ Preparation
ServiceNow instance creation
- You can create an instance for developers by registering an account from the ServiceNow Developers page.
- When the creation is completed, the instance URL and the initial information of the admin account will be displayed, so be sure to make a note of it.
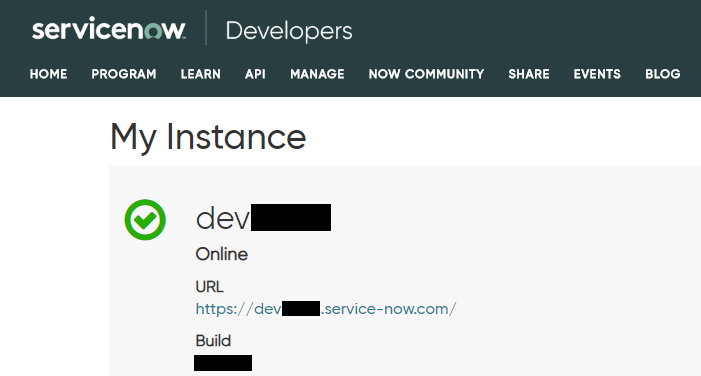
Python execution environment preparation
Preparing Selenium
- Install Selenium with pip.
- Download the driver for the browser you want to run from Selenium official website.
- This time, to run on Google Chrome, download "Google Chrome Driver".
- Place the downloaded "chromedriver.exe" in any location.
◆ Try operating ServiceNow
Launch browser
- Specify the browser as Google Chrome and start it.
- By the way, maximize the window
from selenium import webdriver
#Launch Google Chrome
driver = webdriver.Chrome(executable_path = 'Chrome Driver path')
#Maximize the window
driver.maximize_window()
Access the ServiceNow login page
- Specify the URL for each instance to access.
#Access the ServiceNow login page
driver.get('https://<ServiceNow instance name>.service-now.com/')
driver.implicitly_wait(20)
Get elements of login form
- Since the login form part is in the iframe, if you specify the id as it is, it will not be found and an error will be thrown out.
- It is necessary to get the element of iframe first and move the frame with switch_to_frame.
- When the operation is finished, use switch_to.default_content () to exit the iframe.
#Get the iframe element that contains the login form
login_iframe = driver.find_element_by_xpath("//*[@id='gsft_main']")
#Move into the specified iframe
driver.switch_to_frame(login_iframe)
#Get elements of user name form&Enter user name
user_name_form = driver.find_element_by_id('user_name')
user_name_form.send_keys("<username>")
#Get password form elements&password input
password_form = driver.find_element_by_id('user_password')
password_form.send_keys("<password>")
#Get login button elements&Click login button
login_button = driver.find_element_by_id('sysverb_login')
login_button.click()
driver.implicitly_wait(20)
#Exit the iframe
driver.switch_to.default_content()
◆ Reference page