There was a video of hitting the API on Youtube and it was very easy to understand While doing hands-on, if you don't understand something I will dig deep and write a research article.
I don't need to read anything in particular If you can parse the URL properly, you can use it
ruby 2.5.1p57 Rails 5.2.4.4
API:(Application Programming Interface
)…
Interface → The part that becomes the ** window ** for using the functions of the application
It is usually published on the Web and can be used by anyone for free. You will be able to embed the functions of other software in your own software
API is a function for linking with external applications.
** Hitting the API ** is ... Going to get data using the API The returned data is ** JSON format text **
・ ** Can develop with more new services ** → It is possible to specialize in a certain function, or to make it easier to use and improve only some functions. ・ ** Data can be used secondarily ** → You can use data from other companies and easily analyze the information. ・ ** Can be developed efficiently ** → If the function you want to create is published by API, you do not need to create the same program from scratch ・ ** Improved convenience for service users ** → You can create a function that allows you to log in using the user information of other companies. ・ ** Improved security ** → You can use the system of a company with a high security level
promise
rails new app name
So, create a Searches controller, the action name is "search"
rails g controller searches search
config/routes.rb
Rails.application.routes.draw do
get 'search', to: 'searches#search'
end
Next, modify the view
rb:app/views/layouts/application.html.erb
<!DOCTYPE html>
<html>
<head>
<title>Post code search engine</title>
<%= csrf_meta_tags %>
<%= csp_meta_tag %>
<%= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track': 'reload' %>
<%= javascript_include_tag 'application', 'data-turbolinks-track': 'reload' %>
</head>
<body>
<div class="container">
#Send with get
<%= form_with url: search_path, method: :get, local: true do |f| %>
<%= f.text_field :postal_code, class: "search_form", placeholder: "Please enter your zip code" %>
<% end %>
<%= yield %>
</div>
</body>
</html>
【point】
-The form_with method performs ** ajax asynchronous communication ** by default.
Therefore, HTML will not be rendered without the local: true
option.
-Specify the parameter name in the part of <% = f.text_field: postal_code%>
→ You can get it by typing "params [: postal_encode]
" on the controller.
・ Enter the default characters in the form with placeholder
Next, we will implement the contents of the controller
rb:app/controllers/searches.controller.rb
class SearchsController < ApplicationController
# require 'net/http'
def search
#Get the parameters sent in the form
if postal_code = params[:postal_code]
#Encode here. URI.encode_www_In the form method, "zipcode" at the end of the URL=Making a "zip code"
params = URI.encode_www_form({zipcode: postal_code})
#Get the URL with this, params is a variable so you need to expand it
uri = URI.parse("https://zipcloud.ibsnet.co.jp/api/search?#{params}")
#Getting the response, GET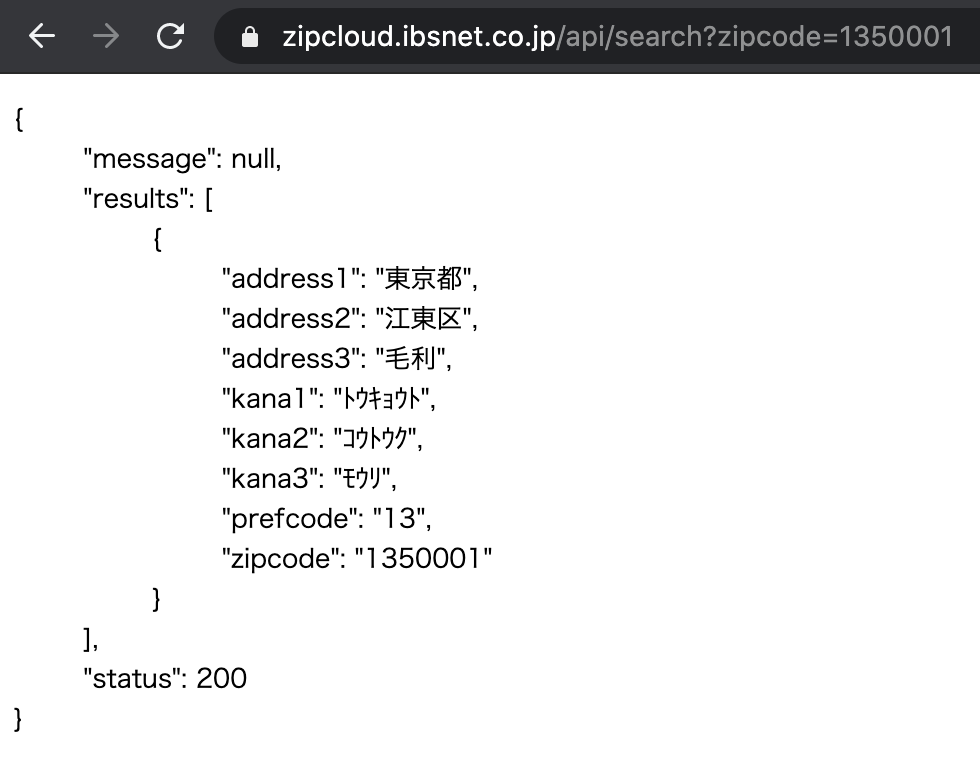
response = Net::HTTP.get_response(uri)
#Convert to JSON format
if result = JSON.parse(response.body)
#Stored in an instance variable for use in view
#Get by specifying the prefecture name and city / ward / town / village in the response
@zipcode = result["results"][0]["zipcode"]
@address1 = result["results"][0]["address1"]
@address2 = result["results"][0]["address2"]
@address3 = result["results"][0]["address3"]
end
end
end
end
** URI.encode_www_form ** method What is encode ... According to certain rules, data in a certain format such as characters Converting to data in another format
[Click here for the completed screen]
Just hit the URL and insert the zip code and you'll get this result
rb:app/searches/search.html.erb
<p>Postal code: <%= @zipcode %></p>
<p>Prefectures: <%= @address1 %></p>
<p>Municipality: <%= @address2 %></p>
<p>Town area: <%= @address3 %></p>
I sometimes got an error,
I solved it by doing " require'net/http'
"on the controller.
Also, if you just want to display it with GET, it seems that you only need to read above (** require **)
https://docs.ruby-lang.org/ja/latest/library/net=2fhttp.html
https://www.youtube.com/watch?v=0fFx9BrP_8g
Recommended Posts