[Python Queue] Convenient use of Deque
Basic operation image of Queue
- Enqueue & Dequeue when the maximum size is 5
- A new element has been added at the end
- The oldest element at the beginning (the element added first) is fetched.
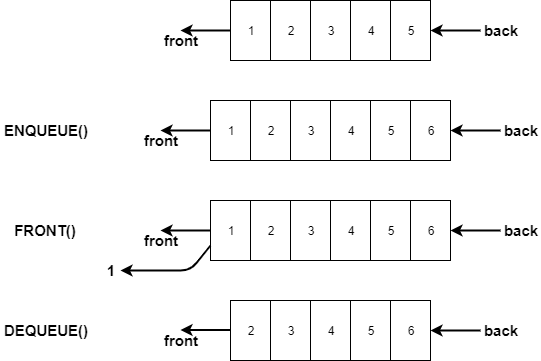
Implementation
- Use deque (Double-Ended Queue). As the name implies, a special queue that allows you to put in and take out data from both sides.
- With only the append method without changing the processing depending on the number of elements in the queue, after reaching the maximum size, the first element is fetched and a new element is added to the end.
from collections import deque
d = deque(maxlen=5)
for i in range(1, 7):
d.append(i)
print(d)
output
deque([1], maxlen=5)
deque([1, 2], maxlen=5)
deque([1, 2, 3], maxlen=5)
deque([1, 2, 3, 4], maxlen=5)
deque([1, 2, 3, 4, 5], maxlen=5) <-Reach maximum size
deque([2, 3, 4, 5, 6], maxlen=5) <- Enqueue &After dequeue
reference