Up to the last time, I was able to learn how to retrieve data and save it in a database, so I would like to develop a web application from this part.
Until last time I want to get League of Legends data ① I want to get League of Legends data ② I want to get League of Legends data ③
As of 2/8/2020 OS:windows10 Anaconda:4.8.1 python:3.7.6 MySQL:8.0.19 Django:3.0.1 PyMySQL:0.9.3
python -m pip install django
Run
Change the library to import from MySQLdb to PyMySQL
python -m pip install pymysql
import mysql -> import pymysql
First, run `django-admin startproject MyProject``` on a directory called APP. 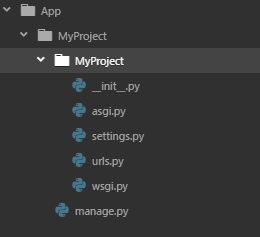 This kind of hierarchy is completed. Execute
python manage.py startapp LOLApp
`` in the same hierarchy as ** manage.py **.
You will have something like this.
*** Added **'LOLApp.apps.LolappConfig', ** to INSTALLED_APPS ***. Then change *** DATABASES ***. Since the database is sqlite3 by default, make it mysql.
Original code
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': os.path.join(BASE_DIR, 'db.sqlite3'),
}
}
After change
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'LOLdb',
'USER': 'root',
'PASSWORD': '{Initial password set}',
'HOST': 'localhost',
'PORT': '3306',
'OPTIONS':{
'init_command': "SET
sql_mode='STRICT_TRANS_TABLES'",
}
}
}
Next, change *** LANGUAGE_CODE *** from **'en-us' ** to **'ja' **. Finally, change *** TIME_ZONE *** from 'UTC' to 'Asia / Tokyo'.
At the very end
import pymysql
pymysql.install_as_MySQLdb
Add.
python manage.Run py makemigrations LOL App
#### **`python manage.Run py migrate`**
```Run py migrate
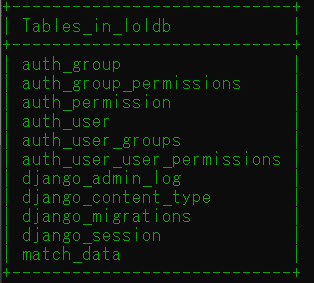
In addition to the previously created table ** match_data **, a new one will be created.
## Start the development server
#### **`python manage.Run py run server and try to jump to the output address.`**
```Run py run server and try to jump to the output address.
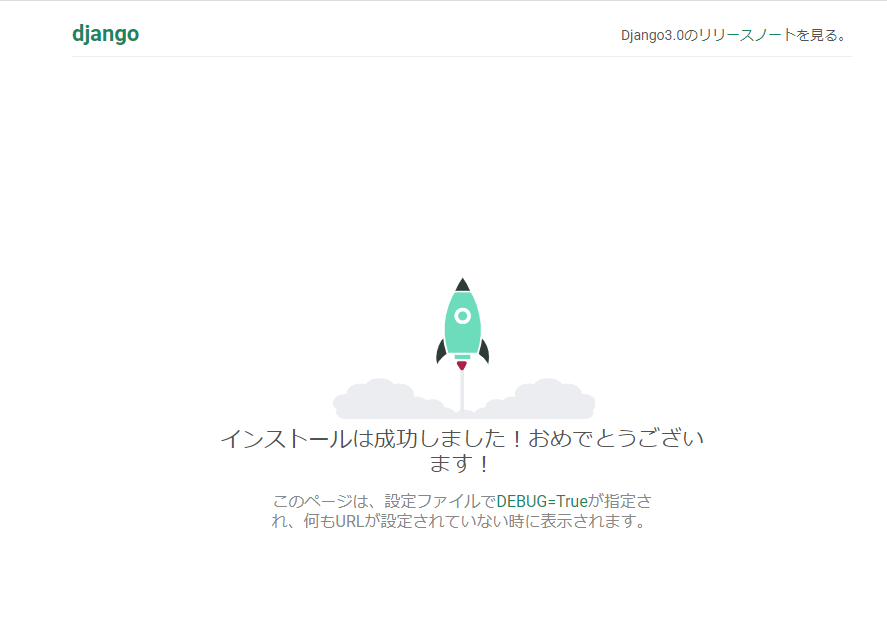
If such a screen appears, it is a success for the time being.
## Create view
#### **`views.py`**
```python
from django.shortcuts import render
from django.http import HttpResponse #add to
# Create your views here.
def index(request):
return HttpResponse("Hello World!")
Create *** urls.py *** directly under *** LOLApp ***. Give the path corresponding to the previous view.
LOLApp/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('',views.index,name='index'),
]
Make changes to *** urls.py *** directly under *** MyProject ***.
MyProject/urls.py
from django.contrib import admin
from django.urls import path,include #add include
urlpatterns = [
path('admin/', admin.site.urls),
path('LOLApp/',include('LOLApp.urls')), #add to
]
python manage.Run py runserver to the previous address**/LOLApp**When you add and access
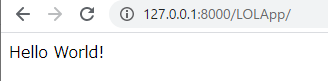
If it is, it is OK for the time being.
### Create a model
#### **`models.py`**
```python
from django.db import models
# Create your models here.
class Match_data(models.Model):
sn = models.CharField(max_length=200)
kills = models.IntegerField(default=0)
deaths = models.IntegerField(default=0)
assists = models.IntegerField(default=0)
championId = models.IntegerField(default=0)
roles = models.CharField(max_length=200)
cs = models.IntegerField(default=0)
gold = models.IntegerField(default=0)
damage = models.IntegerField(default=0)
side = models.CharField(max_length=200)
game_time = models.IntegerField(default=0)
python manage.py makemigrations LOLApp
#### **`python manage.py migrate`**
```py migrate
#### **`python manage.py sqlmigrate LOLApp 0001`**
```py sqlmigrate LOLApp 0001
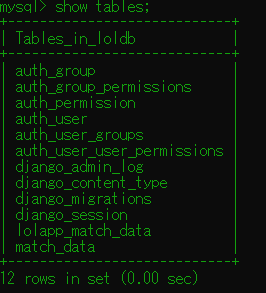
** lolapp_match_data ** is newly added to the table.
#### **`python manage.Run py shell and give it a try.`**
```Run py shell and give it a try.
```python
from LOLApp.models import Match_data
Match_data.objects.all()
# <QuerySet []>
a = Match_data(sn="aaa",kills=2,deaths=3,assists=5,championId=100,roles="MID",cs=100,gold=10000,damage=10000,side="BLUE",game_time=1500)
a.save() #Save data
a
#<Match_data: Match_data object (1)>
If you check with the MySQL command
It was confirmed that it was saved in the database.
python manage.Execute py createsuperuser to register the user name, email address and password.
You can then run ``` python manage.py runsever``` and go to ** http://127.0.0.1:8000/admin/** to jump to the admin site. Modify *** admin.py *** to work with the model on this administration site.
#### **`admin.py`**
```python
from django.contrib import admin
from .models import Match_data
# Register your models here.
admin.site.register(Match_data)
If you add this and reload it
The model has been added
You can work with the data on this screen.
This time, I used the framework to modify the data obtained from the previous item. Next, in order to make a WEB application, I have to make a base to display, so I would like to make that a little.
Recommended Posts