I'm a Rails beginner who creates web apps with Ruby on Rails. Suddenly, I'm sorry to tell you personally, but recently I've been addicted to Youtuber, and I spend most of my time watching Youtube except for studying programming (laughs) YouTube is fun ... YouTuber is good ... Since it is such a daily life, I thought that I would like to make a web application that handles various information on Youtube as an output of study, so I started making it. Great for studying and hobbies! It's simple
While doing a lot of research for that, I found that I needed to use the ** Youtube Data API ** to handle Youtube information.
This time, I would like to write a memorandum about the acquisition of Youtube information using this Youtube API.
This API provides various functions.
・ Resource type
・ Type of operation
This time, I would like to write about what I did to get Youtube channel information. (Resource type: channel, operation: list in the above table)
-You have acquired an API KEY that allows you to access the Youtube Data API by performing various operations with the Google API. Please refer to the following for the procedure of API KEY acquisition.
Search for videos on Youtube using the recent Google API Ruby Client and download them as files [Ruby on Rails] The easiest way to use YouTube API
I am developing Rails by creating a container with Docker for Mac.
ruby 2.4.5
Ruby on rails 5.0.7.2
#Premise gem
#I'm using dotenv to put the GOOGLE API KEY in an environment variable
dotenv-rails 2.7.6
The famous gem for handling this API is the official google google-api-ruby-client
created to hit various Google APIs with rails.
Since there is some information in Japanese and it is official, I was considering this gem at the beginning, but this time I plan to use only the Youtube API, and I thought that I could do it with a simpler one.
That's why I found yt
.
Yt - a Ruby client for the YouTube API
A gem specialized for Youtube API. I was worried because there was not much information and there were few stars, but I was personally an ant because it can be implemented with a simple description! After that, I will write about getting Youtube information with Yt.
First, download the gem. As you can see on yt's github, write it in Gemfile.
Gemfile
gem 'yt', '~> 0.32.0'
Then do a bundle installation.
terminal
bundle install
gemlife.lock
yt (0.32.6)
After downloading yt, write a config that sets the GOOGLE API KEY to yt. When calling various information, only this API KEY is OK because it is only reading the server.
dotenv-rails
.
Reference) How to set environment variablesIf you want to operate a web client such as video upload on Youtube,
You will need client_id
and client_secret
separately from the API KEY.
Reference) Module: Yt :: Config
videos_controller.rb
class VideosController < ApplicationController
GOOGLE_API_KEY = ENV['GOOGLE_API_KEY'] #.How to write when API KEY is already set in env
:
def set_yt
Yt.configure do |config|
config.api_key = GOOGLE_API_KEY #Set google API in Config
end
end
:
end
```
Now, if you call `set_yt` in advance before various operations, the API KEY will be set and you will be able to perform various operations with yt.
# Get Youtube information with yt
This completes the preparations. Let's do various operations immediately.
This time, I will look at two operations that I wanted to do with my web application.
** Part 1) Get the specified number of Youtube subscribers **
** Part 2) Get the video of the specified Youtube channel and save it in the DB **
Let's take a look at each!
## Part 1) Get the specified number of Youtube subscribers
First, bring the Youtube channel ID from the Youtube channel URL.
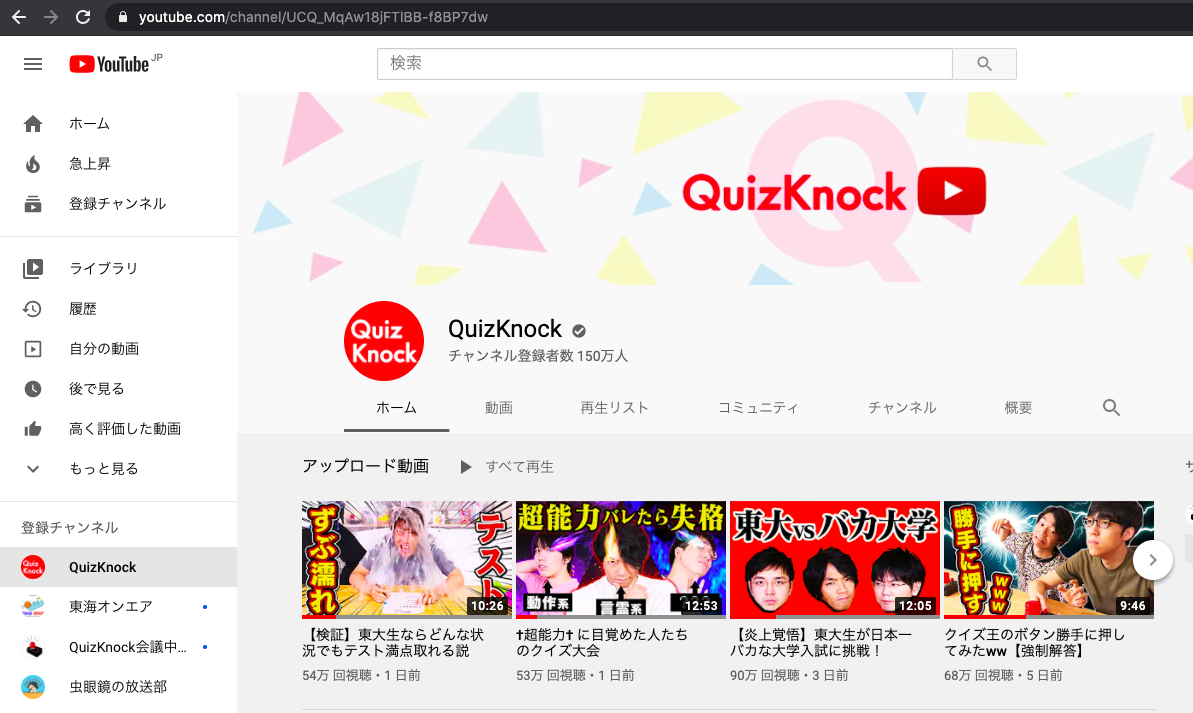
Go to the page of the channel you want to register (screen like the image above), and the part after ** channel / ** of the URL will be the channel ID. In this case, it is the part of ʻUCQ_MqAw18jFTlBB-f8BP7dw`.
We will set this information as the channel id.
Then, the channel information is acquired based on that ID. Very easy.
#### **`videos_controller.rb`**
```ruby
class VideosController < ApplicationController
:
def index #Here is a suitable method
set_yt #Load the config you set earlier
channel = Yt::Channel.new id: 'UCQ_MqAw18jFTlBB-f8BP7dw' #Get channel information
@subscriber_count = channel.subscriber_count #Get the number of registrants
end
:
end
```
Now you can get the number of subscribers by calling `subscriber_count`.
#### **`ruby:index.html.erb`**
```
:
<%= @subscriber_count %>
:
```
It looks like this (I'm processing the way it looks with jquery)
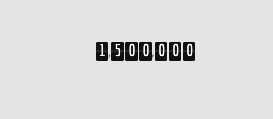
## 2) Get the video of the specified Youtube channel and save it in the DB
Obtaining the channel ID is the same as in Part 1.
In part 2,
① Get channel information
② Get the video associated with that channel
③ Save the information in the DB
There are three main steps.
The save destination table used this time is a video table that holds the video name, URL, and posting date.
If you get all the data at once, you may get caught in the limitation on the Google API side, so I will write to get about 30 most recent videos once.
* See below for how to write in where
(reference)
[I tried using youtube API with Rails](https://qiita.com/sakakinn/items/46c0d4945e4646f346f6)
[I finally found out how to search videos and get multiple information with gem yt! !! ](Https://yatta47.hateblo.jp/entry/2016/10/31/100755)
#### **`videos_controller.rb`**
```ruby
:
#Call youtube video get
def create_videodata
create_videodata_from_youtube
redirect_to videos_path, notice: { "Video acquisition is complete" }
end
:
def create_videodata_from_youtube
set_yt
channel = Yt::channel.new id: 'UCQ_MqAw18jFTlBB-f8BP7dw' #Get channel information
#Get about 30 videos posted in the last 2 months.
channel.videos.where(publisher_afer: 2.months.ago.iso8601).first(30).each do |video|
#If it is not saved video data, add it as a table
unless Video.find_by(name: video.title)
Video.create!(
name: video.title,
url: "https://www.youtube.com/watch?v=" + video.id,
uploaded_at: video.published_at
)
end
end
end
:
```
Now you can get the youtube video by calling the above method when you need it.
In the web application I created, I installed a "Get Video" button and called create_videodata when I pressed it to get Youtube information.
You have now implemented the functionality you wanted to implement. Thanks to Yt, the description is quite simple!
# Troubleshooting (quotaExceeded)
At first, I knew how to write it, but for some reason it didn't work ... When I looked at it, it was "quota Exceeded".
In other words, I was getting an error saying that I used too much and lost the daily allocation, and the Google API was restricted.
At that time, I had never succeeded, so I changed the day thinking, "I can't run out of quotas ...".
I changed my account and recreated it, referring to the following, and it worked!
https://teratail.com/questions/270779
I don't understand why even if I look it up ... If you get the same error, please create a google account and recreate the API KEY with another account.
# At the end
We have seen how to get Youtube information using the Youtube API.
With Yt, it's pretty simple to write, so it's easy to implement what you want to do.
There is a lot of other information I would like to bring, so I would like to investigate and use other functions as well.
Finally, thank you to all the authors of the articles and blogs that I referred to!
Recommended Posts