[Java] Three features of Java
Java features
- Object-orientation
- ** Java Virtual Machine **
- ** Garbage Collection **
Object-oriented thinks about data
- In the first place, the essence is to think about how to process the data and what you want to do.
- In object-oriented programming, the objects handled in the program are represented by objects.
- Everything that makes up an app is represented by objects, and the combination makes up the app.
- ** Capsule / integrate data and its operations and write code **
- A common mistake is to write a method that references data in another object, and a method in another object references data in another object.
- The method of object 1 becomes meaningless → It is called a data object (poor cohesiveness)
- ** The data of object 1 must be referenced by the method of object 1 **
- Basically, let's integrate data and methods!
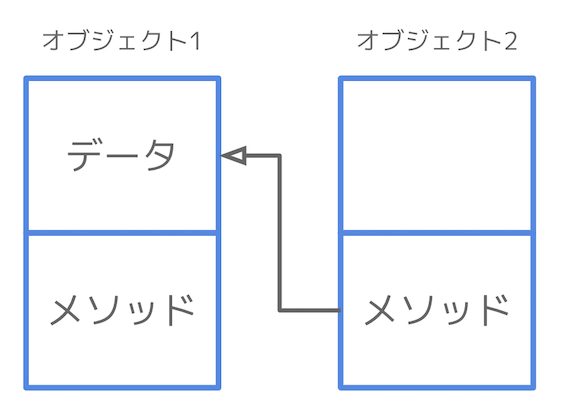
Java virtual machine is binary code
- Java runs on the Java virtual machine
- Java is an improved version of C ++ (which can be said to be), but it has a completely different structure when executed.
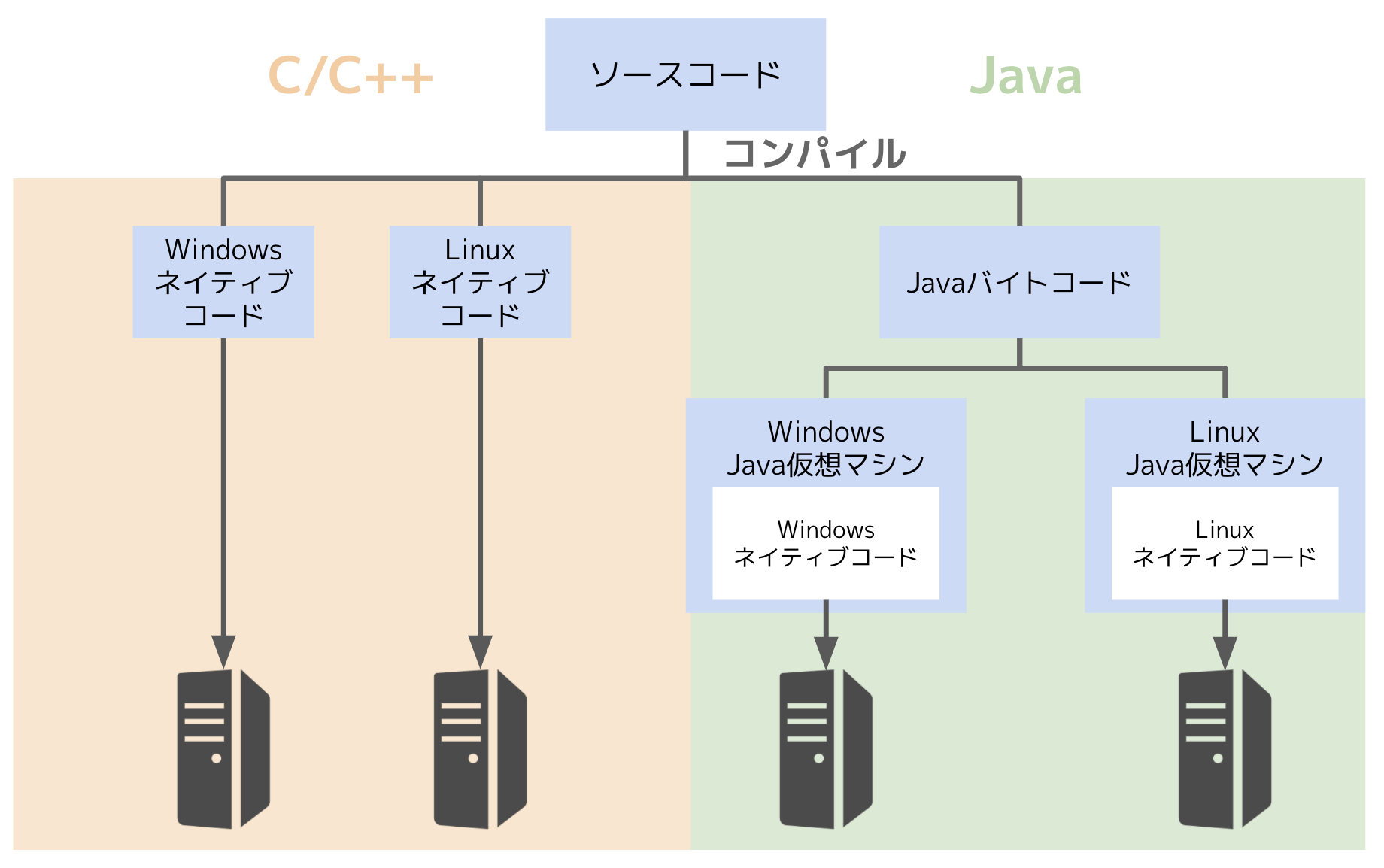
C++
- The PC is powered and the CPU runs
- CPU runs in machine language
- Assembler description (C / C ++) → Compile → Machine language (binary code)
- Assembler directly becomes machine language
- Only binary code can run the CPU directly
Java
- Runs on Java virtual machine
- Create a Java virtual machine on top of the CPU
- Binary code that allows the Java virtual machine to run the CPU directly
- Java virtual machine does not work unless JDK and JRE are installed
- Compiling Java spits out intermediate code
- Intermediate code needs to run on binary code
- It is slower than C ++ that runs the CPU directly because it goes through intermediate code (said)
- Java so it's not slow
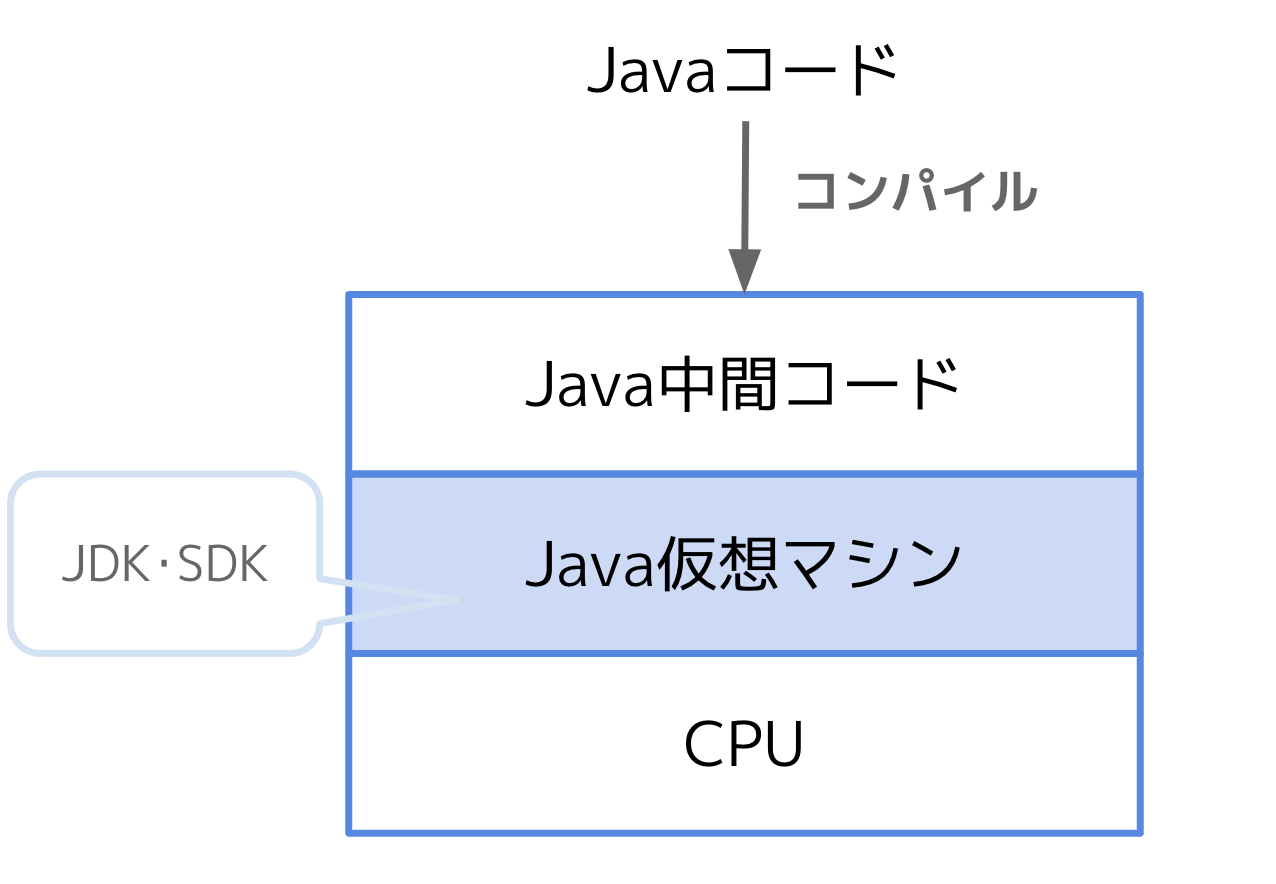
Garbage collection automatically frees memory
CPU / memory (DRAM) / storage (HDD / SSD) configuration
- CPU / DRAM has legs stuck on the board
- Connected by data bus and address bus
- The capacity that DRAM can store is 8 bytes.
- ** Address bus ** signals which address from 0-7 you want to access
- ** Databus ** outputs its contents
- ** CPU / memory is on one foundation **
- Separately from this, it is directly connected
- The address bus has a port called ** I / O port ** that specifies the IO space.
- ** HDD / SSD is connected to the I / O port **
- Storage (HDD / SSD) is not directly connected to the CPU, and DRAM is directly connected.
- The memory of the CPU disappears when the power is not turned on, and the HDD writes to the magnetic material, so it remains even if the electricity is turned off.
- Memory chip type
- ** DRAM **: DDR4 standard, etc. (standard that determines CPU access and latency)
- ** SRAM **: High speed (I don't use it much now)
- ** Flash memory **: Used as external memory (SSD, etc.)
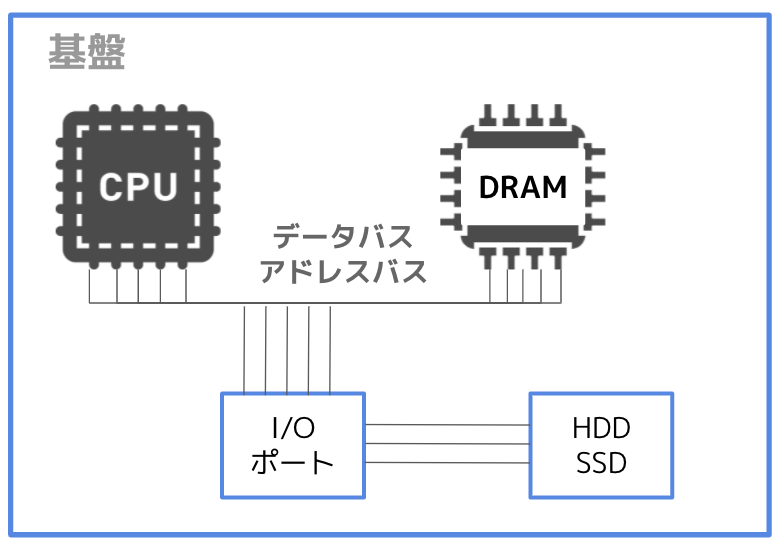
How memory works
- For example, in the case of Z80,
8 data buses + 16 address buses (= indicates the address that the CPU wants to access)
- 0000 0000 0000 0000 ~ 1111 1111 1111 1111 can be accessed
- In the case of Z80 with 8bit CPU, there are ** 0 to 65535 **
- ** Java virtual machine requires OS to use no memory **
- ** OS has a function to manage memory in blocks **
- Java virtual machine requires 100 bytes of memory from the OS
- Return an unused address managed by the OS to the virtual machine
- Java can use that memory
- ** Memory leak **: If a virtual machine is used in a certain application and it dies without telling the end, it will remain used for 100 bytes and cannot be used for other areas.
- In C / C ++, use pointers, allocate using memory allocators, and release with release instructions.
- In Java, the memory provided is under the control of the Java machine of the JDK.
- Write
hoge = null;
or ** the garbage collection will automatically free memory ** when the Java virtual machine is closed.
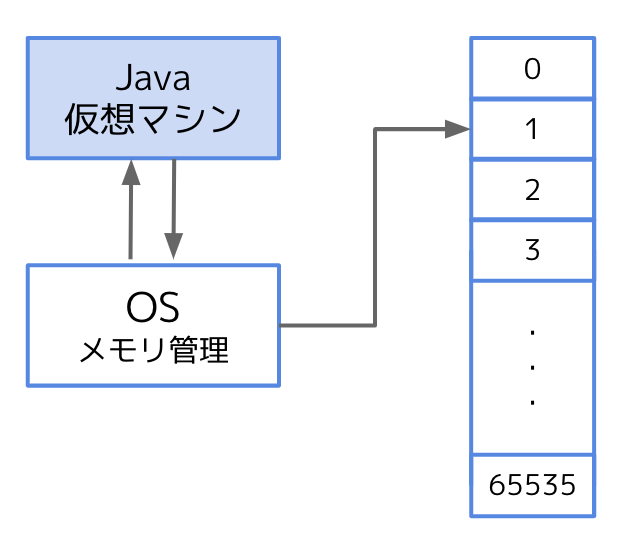