Just try to receive a webhook in ngrok and python
tl;dr
- Sometimes you need a token sent by webhook to create a bot etc.
- Webhook requires a server to publish to the outside, but try to receive the webhook as easily as possible
- Using Python, it was easy to create a server that not only listens but also returns 200 OK.
Subject
- Based on LINE's Messaging API webhook.
- This is JSON format data sent by POST.
- This time, 200 OK is returned so that the LINE side can declare that the webhook can be received.
flow
- Create a server that returns 200 OK no matter what you receive in Python
- Create an environment where you can receive access from the outside with ngrok
- Take a look at the contents of the webhook you received with the Inspect tool that comes with ngrok
Create a server that returns 200 OK no matter what you receive in Python
-
I want to change the behavior according to GET and POST, so I use BaseHTTPRequestHandler of http.server.
- https://docs.python.org/ja/3/library/http.server.html
-
This time, listen on 80 port, and when POST is received, return only 200 OK for the time being.
import http.server
import socketserver
import json
class MyHandler(http.server.BaseHTTPRequestHandler):
def do_POST(self):
self.send_response(200)
self.end_headers()
with socketserver.TCPServer(("", 80), MyHandler) as httpd:
httpd.serve_forever()
- Please execute it after creating it.
serving at port 80
Is displayed, it is OK.
Create an environment where you can receive access from the outside with ngrok
- ngrok is a service that allows you to easily publish your local host to the Internet.
- https://ngrok.com/
- https://qiita.com/mininobu/items/b45dbc70faedf30f484e
- After signing up, the screen below will appear, so follow the guide.
Guide |
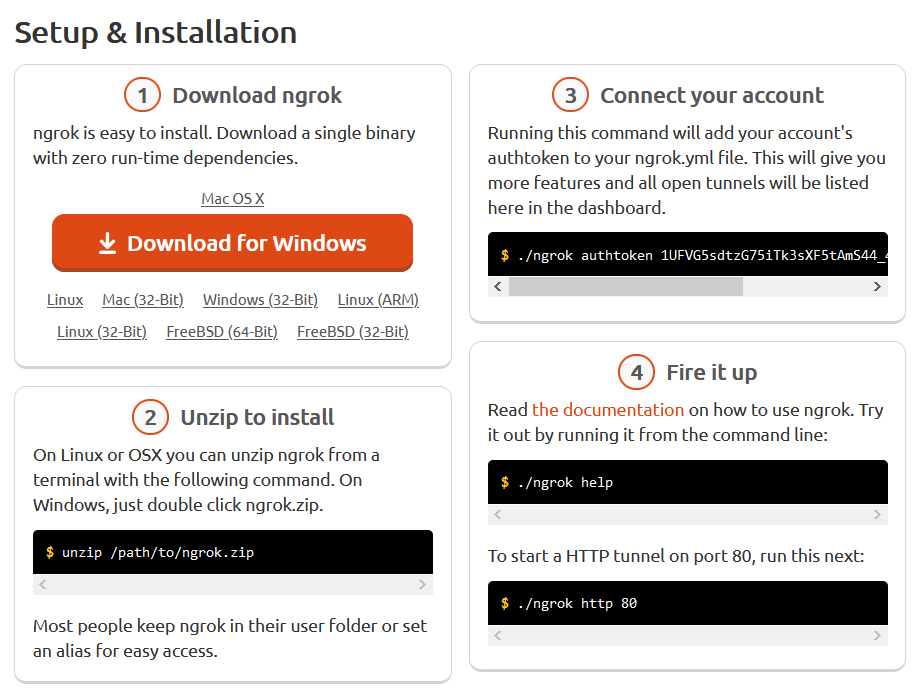 |
- You can register authtoken or start http tunnel at the command prompt, but it is also convenient to use a bat file.
- Unzip the downloaded zip and you will find ngrok.exe.
- Create a file with the following contents in the same location.
auth.bat
ngrok authtoken 1UFVG5sdtzGXXXXXXXXX
80listen.bat
ngrok http 80
- Double-click each to execute.
- auth.bat closes instantly.
- 80listen.bat should look like this:
ngrok |
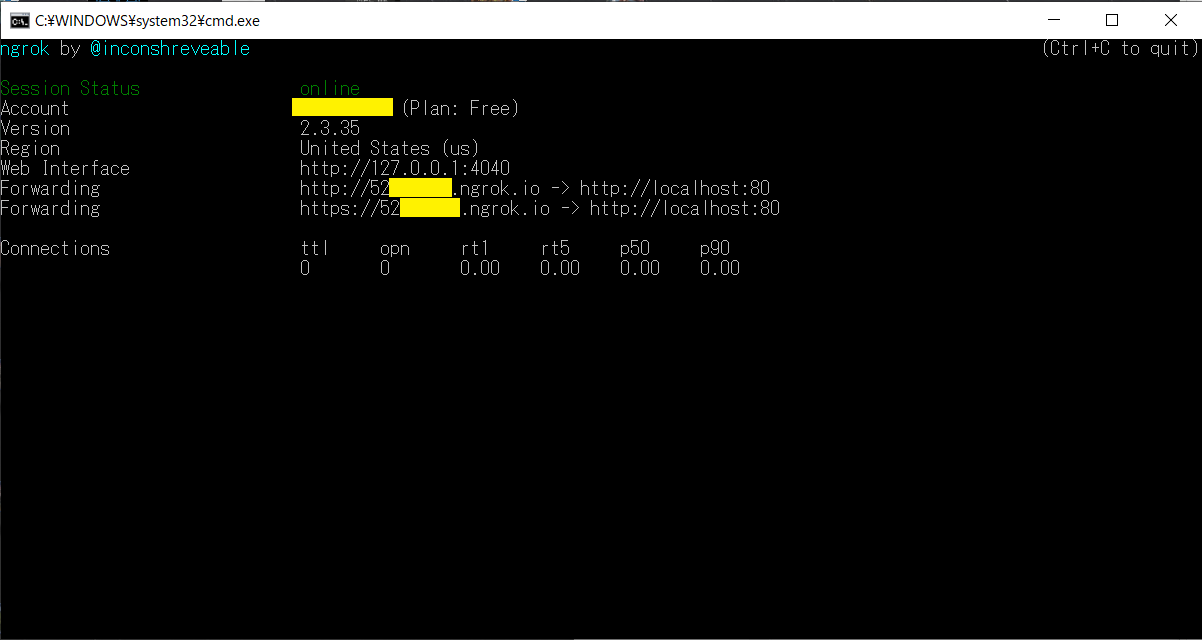 |
- The address ending with ngrok.io in the Forwarding column displayed in this window is your address.
Take a look at the contents of the webhook you received with the Inspect tool that comes with ngrok
- If you try POSTing to this ngrok.io address from the outside
- The ngrok screen shows that POST has arrived at /
ngrok |
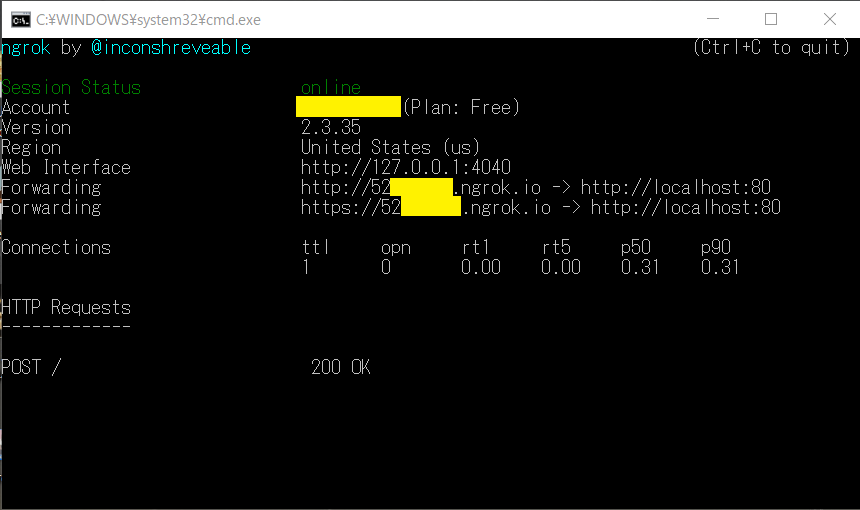 |
-
The contents of the request are displayed on the screen of the server made by python.

-
You can see it more clearly by accessing http: // localhost: 4040 / inspect / http with a browser.
-
https://ngrok.com/docs#getting-started-inspect
inspect screen example |
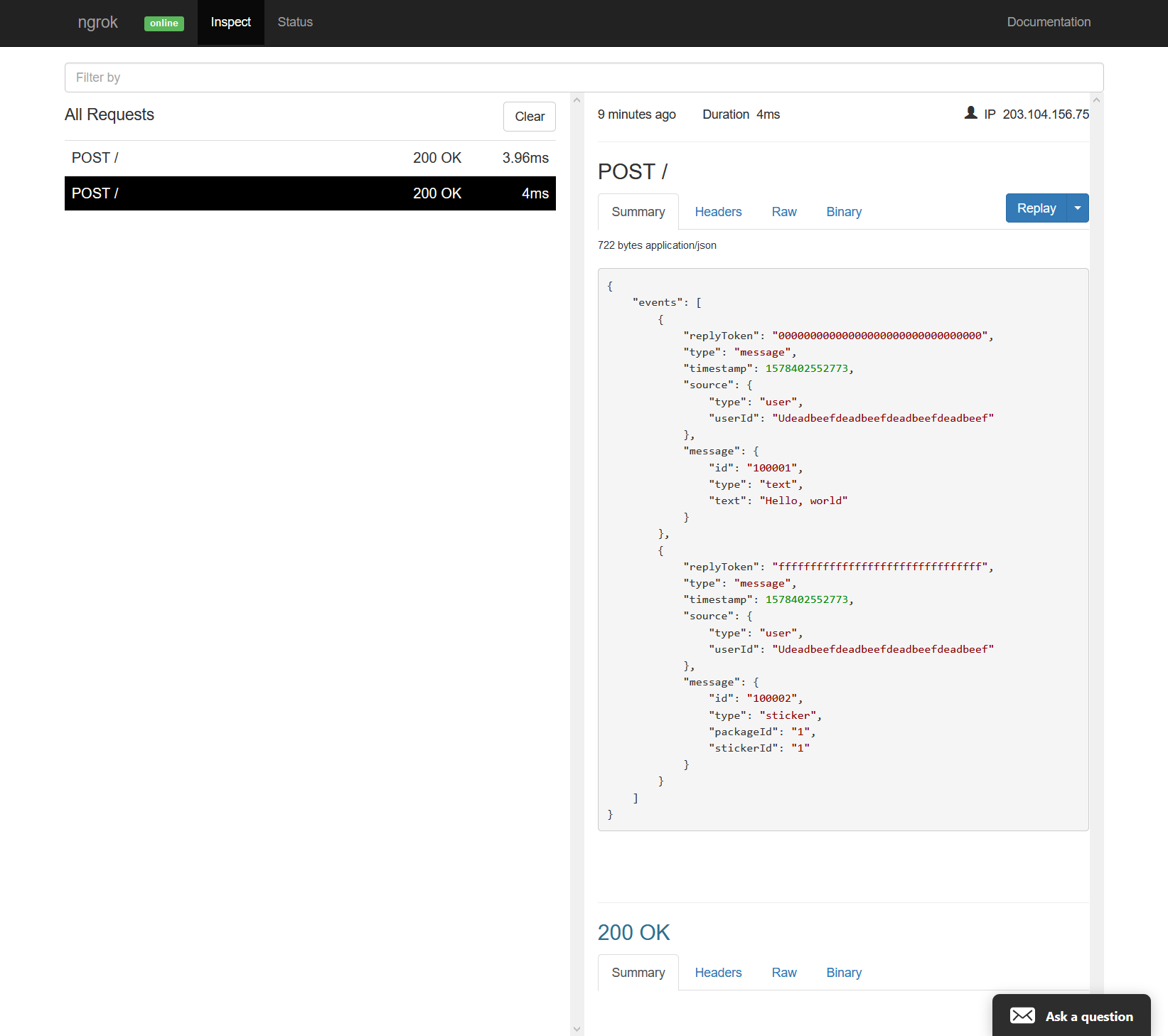 |